Select random numbers items from list in asp.net c#
In this post I will explain about how to Select random numbers items from list in asp.net c# with example.
Description:
In my previous posts I have explained various topics like Sql Server Coalesce,Method Overloading, Abstract Class in C#
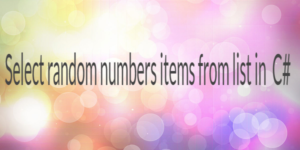
Now in this post, I will explain about how to Select random numbers items from list in asp.net c# with appropriate example.In one of my project I got the requirement to get n random numbers items from list. I was able to get this requirement fulfilled very easily using LINQ. I have made use of LINQ to fulfill this requirement.
Now open Visual Studio , create Console Application and write below line of C#.Net in it.
class Program
{
static void Main(string[] args)
{
List<int> ListOfNumber = new List<int>() { 101, 201, 301, 401, 501, 601, 701, 801, 901, 1001, 1101, 1201 };
List<int> randomNumberList = new List<int>();
randomNumberList = GetRandomElements(ListOfNumber, 5);
foreach (var item in randomNumberList)
{
Console.WriteLine(item);
}
Console.ReadLine();
}
public static List<t> GetRandomElements<t>(IEnumerable<t> list, int elementsCount)
{
return list.OrderBy(x => Guid.NewGuid()).Take(elementsCount).ToList();
}
}
Now as a output you will get any random 5 integer number(list item) from defined Integer List.
Summary
You can also read about ASP.NET, JQuery, JSON, C#.Net
I hope you get an idea about how to generate random numbers items from list in asp.net c#.
I would like to have feedback on my blog.
Your valuable feedback, question, or comments about this article are always welcome.
If you liked this post, don’t forget to share this.