Calculate age from Date of Birth in C#
In this post I will explain about how to Calculate age from Date of Birth in C# with example.
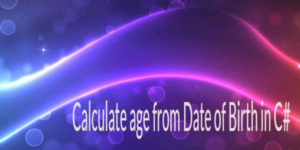
Description:
In my previous posts I have explained various topics like Select random numbers items from list in C#, Sql Server Coalesce,Method Overloading, Abstract Class in C#
Now in this post, I will explain about how to Calculate age from Date of Birth in C# with appropriate example.To achieve this you need to make use of DateTime datatype and related calculation.
Now open Visual Studio , create Console Application and write below line of C#.Net in it.
using LibClass;
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
namespace DotNetByExample
{
class Program
{
static void Main(string[] args)
{
DateTime dob = Convert.ToDateTime("1991/10/28");
string fullAge = CalculateAgeFromDOB(dob);
Console.WriteLine(fullAge);
Console.ReadLine();
}
static string CalculateAgeFromDOB(DateTime p_Dob)
{
// Method to Calculate age from Date of Birth in C#
DateTime Today = DateTime.Now;
int Years = new DateTime(DateTime.Now.Subtract(p_Dob).Ticks).Year - 1;
DateTime PastYearDate = p_Dob.AddYears(Years);
int Months = 0;
for (int i = 1; i <= 12; i++)
{
if (PastYearDate.AddMonths(i) == Today)
{
Months = i;
break;
}
else if (PastYearDate.AddMonths(i) >= Today)
{
Months = i - 1;
break;
}
}
int Days = Today.Subtract(PastYearDate.AddMonths(Months)).Days;
int Hours = Today.Subtract(PastYearDate).Hours;
int Minutes = Today.Subtract(PastYearDate).Minutes;
int Seconds = Today.Subtract(PastYearDate).Seconds;
return String.Format("Age: {0} Year(s) {1} Month(s) {2} Day(s) {3} Hour(s) {4} Second(s)",
Years, Months, Days, Hours, Seconds);
}
}
}
When you run above code snippet you will below output.
Age: 28 Year(s) 7 Month(s) 10 Day(s) 20 Hour(s) 19 Second(s)
Summary
You can also read about ASP.NET, JQuery, JSON, C#.Net
I hope you get an idea about how to Calculate age from DOB in C#.
I would like to have feedback on my blog.
Your valuable feedback, question, or comments about this article are always welcome.
If you liked this post, don’t forget to share this.